Working with strings
String operations
Strings can be combined, or concatenated, with the +
operator:
begin = "ex"
end = "ample"
word = begin+end
print(word)
example
The *
operator can also be used with a string, when the other operand is an integer. The string operand is then repeated the number of times specified by the integer. For example this would work:
word = "banana"
print(word*3)
bananabananabanana
Using string operations together with a loop we can write a program which draws a pyramid:
n = 10 # number of layers in the pyramid
row = "*"
while n > 0:
print(" " * n + row)
row += "**"
n -= 1
This prints out the following:
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
The print
command within the loop prints a line, which begins with n
spaces, followed by whatever is stored in the variable row
. Then two stars are added to the end of the variable row
, and the value of the variable n
is decreased by 1.
The length and index of a string
The function len
returns the number of characters in a string, which is always an integer value. For example, len("hey")
returns 3, because there are three characters in the string hey
.
The following program asks the user for a string and then prints it "underlined". The program prints a second line with as many -
characters as is the length of the input:
input_string = input("Please type in a string: ")
print(input_string)
print("-"*len(input_string))
Please type in a string: Hi there!
Hi there! ---------
The length of a string includes all the characters in the string, including whitespace. For example, the length of the string bye bye
is 7.
As strings are essentially sequences of characters, any single character in a string can also be retrieved. The operator []
finds the character with the index specified within the brackets.
The index refers to a position in the string, counting up from zero. The first character in the string has index 0, the second character has index 1, and so forth.
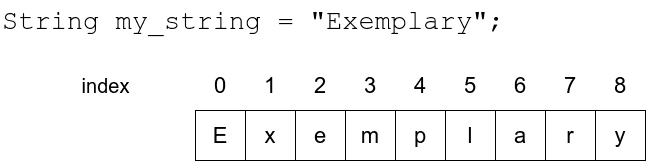
For example, this program
input_string = input("Please type in a string: ")
print(input_string[0])
print(input_string[1])
print(input_string[3])
would print out this:
Please type in a string: monkey m o k
Since the first character in a string has the index 0, the last character has the index length - 1. The following program prints out the first and the last characters of a string:
input_string = input("Please type in a string: ")
print("First character: " + input_string[0])
print("Last character: " + input_string[len(input_string) - 1])
Please type in a string: testing First character: t Last character: g
The following program loops through all the characters in a string from first to last:
input_string = input("Please type in a string: ")
index = 0
while index < len(input_string):
print(input_string[index])
index += 1
Please type in a string: test t e s t
You can also use negative indexing to access characters counting from the end of the string. The last character in a string is at index -1, the second to last character is at index -2, and so forth. You can think of input_string[-1]
as shorthand for input_string[len(input_string) - 1]
.
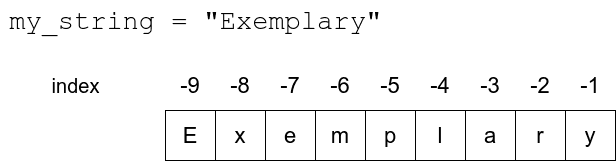
The example from above can be simplified with negative indexing:
input_string = input("Please type in a string: ")
print("First character: " + input_string[0])
print("Last character: " + input_string[-1])
Please type in a string: testing First character: t Last character: g
IndexError: string index out of range
If you tried the above examples for yourself, you may already have come across the error message IndexError: string index out of range. This error appears if you try to access an index which is not present in the string.
input_string = input("Please type in a string: ")
print("The tenth character: " + input_string[9])
Please type in a string: introduction to programming The tenth character: i
Please type in a string: python
Sometimes an indexing error is caused by a bug in the code. For example, it is quite common to index too far when trying to access the last character in a string:
input_string = input("Please type in a string: ")
print("Last character: " + input_string[len(input_string)])
Since string indexing begins at zero, the last character is at index len(input_string) - 1
, not at len(input_string)
.
There are situations where the program should prepare for errors caused by input from the user:
input_string = input("Please type in a string: ")
if len(input_string) > 0:
print("First character: " + input_string[0])
else:
print("The input string is empty. There is no first character.")
In the example above, if the programmer hadn't included a check for the length of the input string, a string of length zero would have caused an error. A string of length zero is also called an empty string, and here it would be achieved by just pressing Enter at the input prompt.
Substrings and slices
A substring of a string is a sequence of characters that forms a part of the string. For example, the string example
contains the substrings exam
, amp
and ple
, among others. In Python programming, the process of selecting substrings is usually called slicing, and a substring is often referred to as a slice of the string. The two terms can often be used interchangeably.
If you know the beginning and end indexes of the slice you wish to extract, you can do so with the notation [a:b]
. This means the slice begins at the index a
and ends at the last character before index b
- that is, including the first, but excluding the last. You can think of the indexes as separator lines drawn on the left side of the indexed character, as illustrated in the image below:
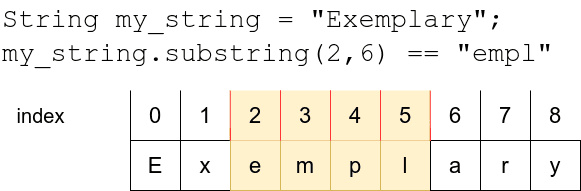
Let's have a closer look at some sliced strings:
input_string = "presumptious"
print(input_string[0:3])
print(input_string[4:10])
# if the beginning index is left out, it defaults to 0
print(input_string[:3])
# if the end index is left out, it defaults to the length of the string
print(input_string[4:])
pre umptio pre umptious
Searching for substrings
The in
operator can tell us if a string contains a particular substring. The Boolean expression a in b
is true, if b
contains the substring a
.
For example, this bit of code
input_string = "test"
print("t" in input_string)
print("x" in input_string)
print("es" in input_string)
print("ets" in input_string)
prints out the following:
True False True False
The program below lets the user search for substrings within a string hardcoded into the program:
input_string = "perpendicular"
while True:
substring = input("What are you looking for? ")
if substring in input_string:
print("Found it")
else:
print("Not found")
What are you looking for? perp Found it What are you looking for? abc Not found What are you looking for? pen Found it ...
The operator in
returns a Boolean value, so it will only tell us if a substring exists in a string, but it will not be useful in finding out where exactly it is. Instead, the Python string method find
can be used for this purpose. It takes the substring searched for as an argument, and returns either the first index where it is found, or -1
if the substring is not found within the string.
The image below illustrates how it is used:
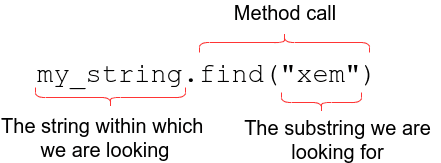
Some examples of how find
is used:
input_string = "test"
print(input_string.find("t"))
print(input_string.find("x"))
print(input_string.find("es"))
print(input_string.find("ets"))
0 -1 1 -1
The above substring search example implemented with find
:
input_string = "perpendicular"
while True:
substring = input("What are you looking for? ")
index = input_string.find(substring)
if index >= 0:
print(f"Found it at the index {index}")
else:
print("Not found")
What are you looking for? perp Found it at the index 0 What are you looking for? abc Not found What are you looking for? pen Found it at the index 3 ...
You can check your current points from the blue blob in the bottom-right corner of the page.